Introduction Last updated: 2023-07-27
At Centralisera Limited, we're dedicated to providing you with seamless consolidation of your payment stack and creating a great payment experience. Our API is designed to make integration effortless, enabling your customer to be redirected to our payment page without a hitch.
Don't hesitate to reach out with any questions or feedback.
Make Payment Request
Name: Payment Request
Method : POST
Url : {{BASE_URL}}/api/v1/payment/request
Authorization
Basic Auth
Username
Password
Body
Raw JSON
{
"firstName" : "John",
"middleName" : "", //optional
"lastName" : "Doe",
"reference" : "Test123456", //would be 10 characters and more and special characters not allowed
"dob" : "2023-07-20",
"email" : "[email protected]",
"contactNumber" : "+889943432",
"address" : "Sweden",
"country" : "Sweden",
"state" : "Blekinge", //If don't have any city, use "N/A" or "country_name"
"city" : "Jämshög",
"zipCode" : 11115,
"currency" : "USD", //ISO 4217 format
"amount" : 110,
"ttl" : 5, //optional (default value : 15 minutes)
"tagName" : "macbook pro m2", //optional
"merchantAccountId" : "100001",
"webhookUrl" : "https://example.webhook", //optional (if your Api Config already have ipn-url set &
if you send webhookUrl in the payload then it will be overwrite your config ipn & webhook url.)
}
N:B: If your country is missing from the list, please contact the help panel to activate.
Response Example
JSON
{
"success" : True,
"message" : "Payment Request Accepted",
"data" :{"payment_url" : "https://staging.centralisera.com/secure-payment/2bfec39f-55dc-4f48-976b-9d37b1675007"},
}
Dynamic Currency Payment By Cashier
Name: Dynamic Currency Payment
Method : POST
Url : {{BASE_URL}}/api/v1/payment/request
Description : If you need to convert currency to a different currency then you have to add another field depositCurrency which will be converted from the base currency. For example - if you deposit 100 USD to GBP then 79.45 GBP will be deposited
Authorization
Basic Auth
Username
Password
Body
Raw JSON
{
"firstName" : "John",
"middleName" : "", //optional
"lastName" : "Doe",
"reference" : "Test123456", //would be 10 characters and more and special characters not allowed
"dob" : "2023-07-20",
"email" : "[email protected]",
"contactNumber" : "+889943432",
"address" : "Sweden",
"country" : "Sweden",
"state" : "Blekinge", //If don't have any city, use "N/A" or "country_name"
"city" : "Jämshög",
"zipCode" : 11115,
"currency" : "USD", //ISO 4217 format
"depositCurrency" : "GBP", //optional - ISO 4217 format
"amount" : 110,
"ttl" : 5, //optional (default value : 15 minutes)
"tagName" : "macbook pro m2", //optional
"merchantAccountId" : "100001",
"webhookUrl" : "https://example.webhook", //optional (if your Api Config already have ipn-url set &
if you send webhookUrl in the payload then it will be overwrite your config ipn & webhook url.)
}
N:B: If your country is missing from the list, please contact the help panel to activate.
Response Example
JSON
{
"success" : True,
"message" : "Payment Request Accepted",
"data" :{"payment_url" : "https://staging.centralisera.com/secure-payment/2bfec39f-55dc-4f48-976b-9d37b1675007"},
}
Country List
Name: Get Country List
Method : GET
Url : {{BASE_URL}}/api/v1/country-list?merchantAccountId={your_account_no}
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Param
merchantAccountId : your_account_no
Response Example
JSON
{
"success" : True,
"message" : "Country list",
"data" :[
{
"id": 213,
"name": "Sweden",
"emoji": "🇸🇪",
"flag": null,
"maps": [
{
"country": "",
"solution": "",
"solution_id": ""
}
],
"name_alpha_2": "SE",
"name_alpha_3": "SWE",
"numeric_code": "752"
},
] , // array of list
}
State List by Country id
Name: Get State List by Country id
Method : GET
Url : {{BASE_URL}}/api/v1/states-by-country/{country_id}?merchantAccountId={your_account_no}
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Param
merchantAccountId : your_account_no
Response Example
JSON
{
"success" : True,
"message" : "State list",
"data" :[
{
"id": 1533,
"name": "Gävleborg County",
"country_code": "SE",
"iso2": "X"
},
] , // array of list
}
Cities List by State id
Name: Get Cities List by State id
Method : GET
Url : {{BASE_URL}}/api/v1/cities-by-state/{state_id}?merchantAccountId={your_account_no}
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Param
merchantAccountId : your_account_no
Response Example
JSON
{
"success" : True,
"message" : "Cities list",
"data" :[
{
"id": 1533,
"name": "Gävleborg County",
"state_code": "X",
"country_code": "SE"
},
] , // array of list
}
API Configuration
This section reading about Centralisera APi Configuration.
Basic Auth
Username & Password :
You can use "username" as "App key" and "password" will be "Secrete Key" from the "Api Config" menu.
See the attached image
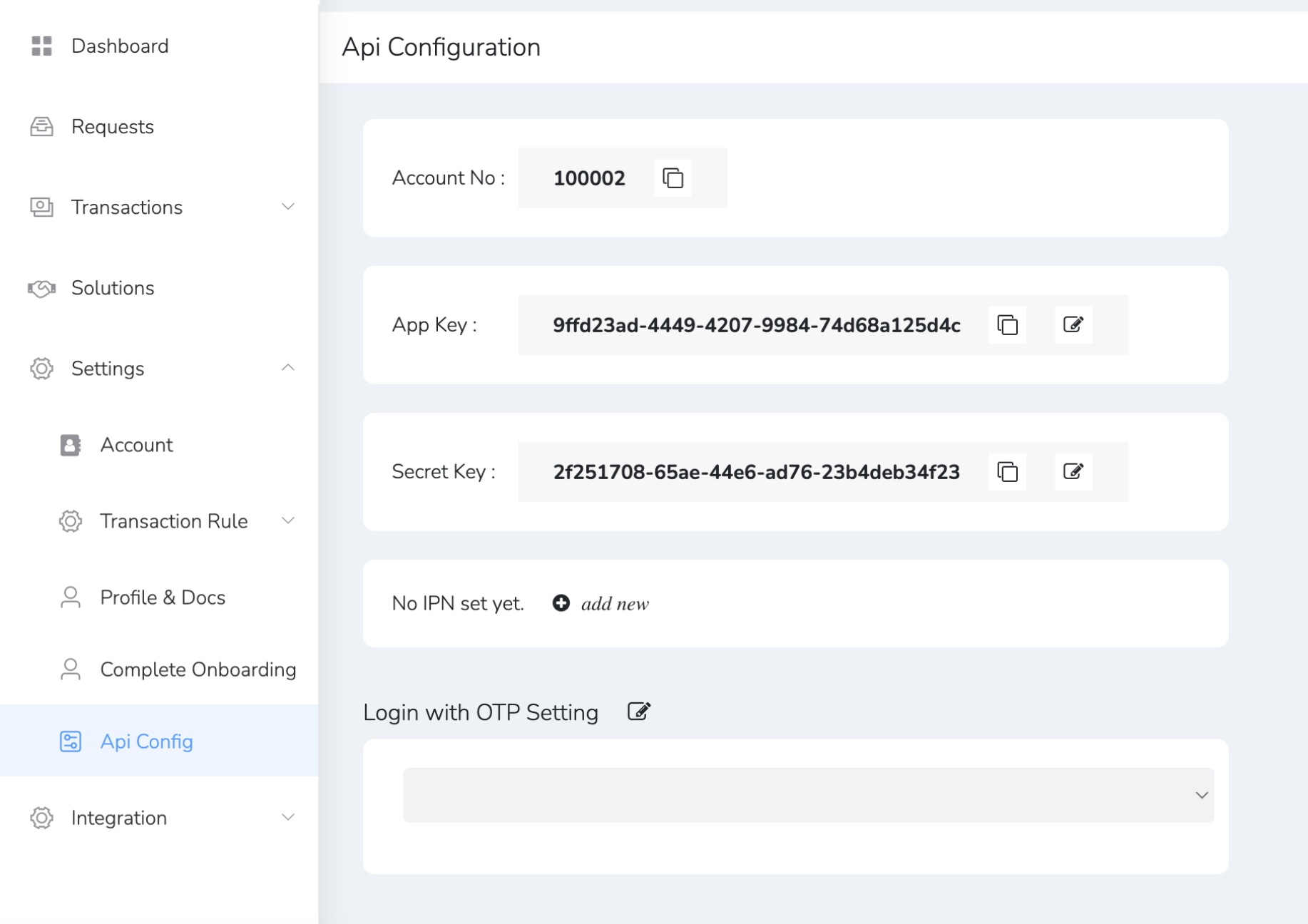
IPN & Webhook Setting
"IPN Url" This URL is used to receive hook data on new transaction complete or failure notifications.
You can set the IPN URL from Settings -> API Config.
If you wish to overwrite the Config you can also send webhookUrl url via the payment request payload (check payment request menu).
"Webhook Url" for getting transactions update Notification hook data.
Response Example
JSON
{
"type": "sale", //refund,payout
"amount": "24.00",
"status": "success", //success or failed
"reference": "O4j2V5thWT",
"transaction_id": "56-9-3485176",
"created_at": "2024-01-19T10:49:27.000000Z",
"payment_method": "Card (VISA)",
"processor_name": "Trust Payments",
"currency": "USD",
}
See the attached image
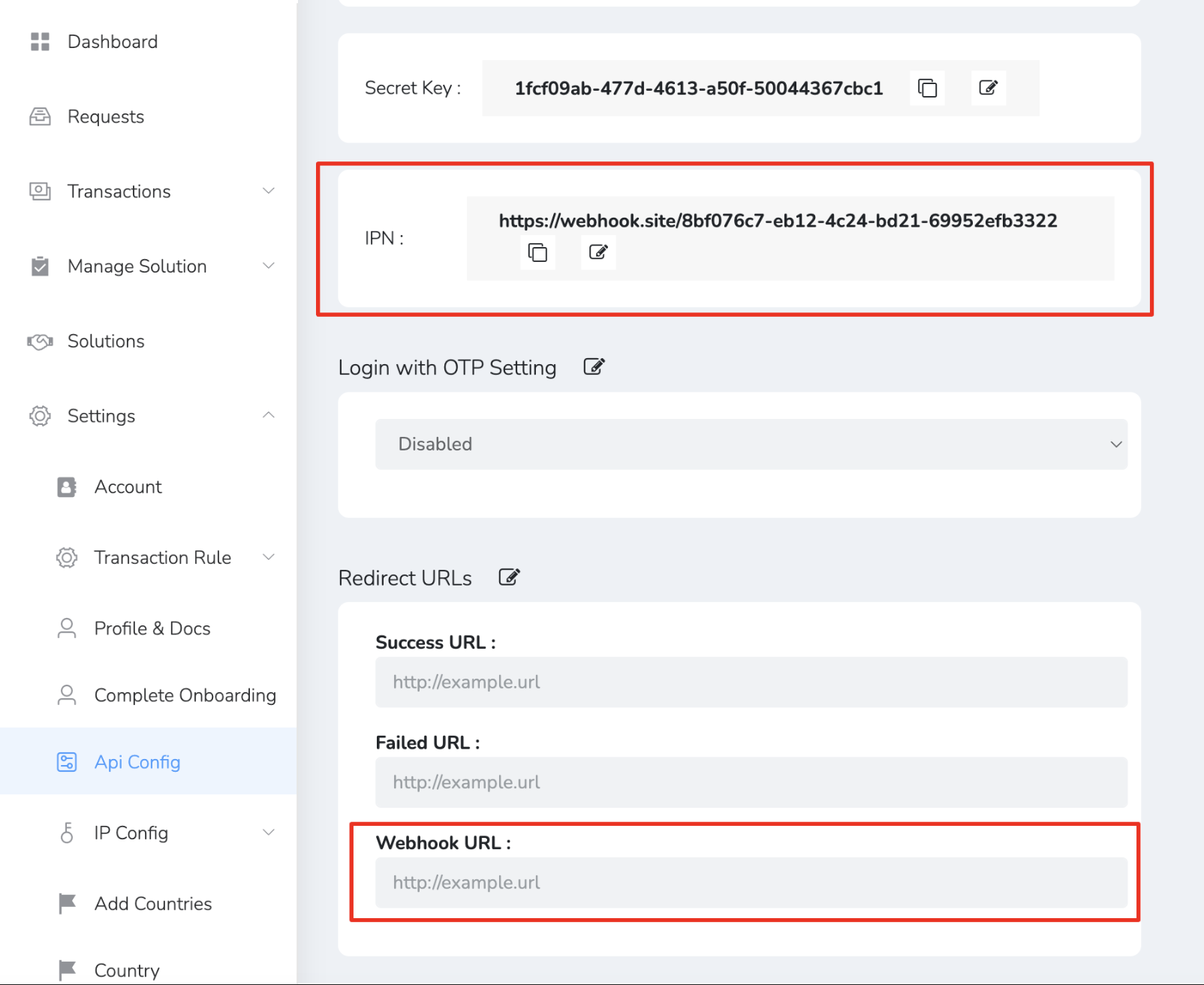
Verify Webhook with Signature
To ensure the integrity of webhook data, every webhook request includes a digital signature in the header. You should verify this signature using your Secrete Key before processing the request.
Signature Verification Steps
- Convert the payload into a JSON string (ensure proper formatting).
- In header, you will get the signature.
- Retrieve the JSON payload received from the webhook request.
- Alphabetically sort the payload before generating the signature.
- Generate an HMAC-SHA256 hash using the Settings -> Api Config -> Secrete Key as the apiSecret variable's value.
- Compare the generated signature with the signature received in the request headers.
// Payload received from webhook
$payload = [
"status" => "failed",
"type" => "sale",
"amount" => "55.00",
"reference" => "DazuknyVyG",
"transaction_id" => "A49dfkqvw",
"payment_method" => "Card (VISA)",
"created_at" => "2025-02-25 05:18:56",
"processor_name" => "Trust Payments",
"currency" => "USD"
];
// Fetch API Secret Text from settings/config
$apiSecret = getenv('API_SECRET') ?: 'default_secret'; // Replace with your actual config fetching logic
// Received signature (from the request headers or body)
$receivedSignature = "your_received_signature_here"; // Replace with the actual signature
// Step 1: Sort the payload by keys alphabetically
ksort($payload);
// Step 2: Convert payload to JSON string
$payloadJson = json_encode($payload, JSON_UNESCAPED_SLASHES | JSON_UNESCAPED_UNICODE);
// Step 3: Generate HMAC signature using SHA-256
$generatedSignature = hash_hmac('sha256', $payloadJson, $apiSecret);
// Step 4: Verify the signature
if (hash_equals($generatedSignature, $receivedSignature)) {
echo "Signature is valid!";
} else {
echo "Signature is invalid!";
}
const crypto = require('crypto');
// Payload received from webhook
let payload = {
status: "failed",
type: "sale",
amount: "55.00",
reference: "DazuknyVyG",
transaction_id: "A49dfkqvw",
payment_method: "Card (VISA)",
created_at: "2025-02-25 05:18:56",
processor_name: "Trust Payments",
currency: "USD"
};
// Fetch API Secret Text from settings/config
const apiSecret = process.env.API_SECRET || 'default_secret'; // Replace with your actual config fetching logic
// Received signature (from the request headers or body)
const receivedSignature = "your_received_signature_here";
// Step 1: Sort the payload by keys alphabetically
payload = Object.keys(payload).sort().reduce((obj, key) => {
obj[key] = payload[key];
return obj;
}, {});
// Step 2: Convert payload to JSON string
const payloadJson = JSON.stringify(payload);
// Step 3: Generate HMAC signature using SHA-256
const generatedSignature = crypto.createHmac('sha256', apiSecret)
.update(payloadJson)
.digest('hex');
// Step 4: Verify the signature
if (generatedSignature === receivedSignature) {
console.log("Signature is valid!");
} else {
console.log("Signature is invalid!");
}
import hmac
import hashlib
import json
import os
# Payload received from webhook
payload = {
"status": "failed",
"type": "sale",
"amount": "55.00",
"reference": "DazuknyVyG",
"transaction_id": "A49dfkqvw",
"payment_method": "Card (VISA)",
"created_at": "2025-02-25 05:18:56",
"processor_name": "Trust Payments",
"currency": "USD"
}
# Fetch API Secret Text from settings/config
api_secret = os.getenv('API_SECRET', 'default_secret') # Replace with your actual config fetching logic
# Received signature (from the request headers or body)
received_signature = "your_received_signature_here"
# Step 1: Sort the payload by keys alphabetically
sorted_payload = dict(sorted(payload.items()))
# Step 2: Convert payload to JSON string
payload_json = json.dumps(sorted_payload, separators=(',', ':'))
# Step 3: Generate HMAC signature using SHA-256
generated_signature = hmac.new(
api_secret.encode('utf-8'),
payload_json.encode('utf-8'),
hashlib.sha256
).hexdigest()
# Step 4: Verify the signature
if hmac.compare_digest(generated_signature, received_signature):
print("Signature is valid!")
else:
print("Signature is invalid!")
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security.Cryptography;
using System.Text;
using Newtonsoft.Json;
class Program
{
static void Main()
{
// Payload received from webhook
var payload = new Dictionary
{
{ "status", "failed" },
{ "type", "sale" },
{ "amount", "55.00" },
{ "reference", "DazuknyVyG" },
{ "transaction_id", "A49dfkqvw" },
{ "payment_method", "Card (VISA)" },
{ "created_at", "2025-02-25 05:18:56" },
{ "processor_name", "Trust Payments" },
{ "currency", "USD" }
};
// Fetch API Secret Text from settings/config
string apiSecret = Environment.GetEnvironmentVariable("API_SECRET") ?? "default_secret"; // Replace with your actual config fetching logic
// Received signature (from the request headers or body)
string receivedSignature = "your_received_signature_here";
// Step 1: Sort the payload by keys alphabetically
var sortedPayload = payload.OrderBy(kvp => kvp.Key).ToDictionary(kvp => kvp.Key, kvp => kvp.Value);
// Step 2: Convert payload to JSON string
string payloadJson = JsonConvert.SerializeObject(sortedPayload);
// Step 3: Generate HMAC signature using SHA-256
using (var hmac = new HMACSHA256(Encoding.UTF8.GetBytes(apiSecret)))
{
byte[] hashBytes = hmac.ComputeHash(Encoding.UTF8.GetBytes(payloadJson));
string generatedSignature = BitConverter.ToString(hashBytes).Replace("-", "").ToLower();
// Step 4: Verify the signature
if (generatedSignature == receivedSignature)
{
Console.WriteLine("Signature is valid!");
}
else
{
Console.WriteLine("Signature is invalid!");
}
}
}
}
import java.nio.charset.StandardCharsets;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.util.*;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import com.fasterxml.jackson.databind.ObjectMapper;
public class SignatureVerifier {
public static void main(String[] args) throws Exception {
// Payload received from webhook
Map payload = new HashMap<>();
payload.put("status", "failed");
payload.put("type", "sale");
payload.put("amount", "55.00");
payload.put("reference", "DazuknyVyG");
payload.put("transaction_id", "A49dfkqvw");
payload.put("payment_method", "Card (VISA)");
payload.put("created_at", "2025-02-25 05:18:56");
payload.put("processor_name", "Trust Payments");
payload.put("currency", "USD");
// Fetch API Secret Text from settings/config
String apiSecret = System.getenv("API_SECRET") != null ? System.getenv("API_SECRET") : "default_secret"; // Replace with your actual config fetching logic
// Received signature (from the request headers or body)
String receivedSignature = "your_received_signature_here";
// Step 1: Sort the payload by keys alphabetically
Map sortedPayload = new TreeMap<>(payload);
// Step 2: Convert payload to JSON string
ObjectMapper objectMapper = new ObjectMapper();
String payloadJson = objectMapper.writeValueAsString(sortedPayload);
// Step 3: Generate HMAC signature using SHA-256
Mac sha256_HMAC = Mac.getInstance("HmacSHA256");
SecretKeySpec secret_key = new SecretKeySpec(apiSecret.getBytes(StandardCharsets.UTF_8), "HmacSHA256");
sha256_HMAC.init(secret_key);
byte[] hashBytes = sha256_HMAC.doFinal(payloadJson.getBytes(StandardCharsets.UTF_8));
String generatedSignature = bytesToHex(hashBytes);
// Step 4: Verify the signature
if (generatedSignature.equals(receivedSignature)) {
System.out.println("Signature is valid!");
} else {
System.out.println("Signature is invalid!");
}
}
private static String bytesToHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
}
package main
import (
"crypto/hmac"
"crypto/sha256"
"encoding/hex"
"encoding/json"
"fmt"
"os"
"sort"
)
func main() {
// Payload received from webhook
payload := map[string]string{
"status": "failed",
"type": "sale",
"amount": "55.00",
"reference": "DazuknyVyG",
"transaction_id": "A49dfkqvw",
"payment_method": "Card (VISA)",
"created_at": "2025-02-25 05:18:56",
"processor_name": "Trust Payments",
"currency": "USD",
}
// Fetch API Secret Text from settings/config
apiSecret := os.Getenv("API_SECRET")
if apiSecret == "" {
apiSecret = "default_secret" // Replace with your actual config fetching logic
}
// Received signature (from the request headers or body)
receivedSignature := "your_received_signature_here" // Replace with the actual signature
// Step 1: Sort the payload by keys alphabetically
keys := make([]string, 0, len(payload))
for k := range payload {
keys = append(keys, k)
}
sort.Strings(keys)
sortedPayload := make(map[string]string, len(payload))
for _, k := range keys {
sortedPayload[k] = payload[k]
}
// Step 2: Convert payload to JSON string
payloadJSON, err := json.Marshal(sortedPayload)
if err != nil {
fmt.Println("Error marshalling payload:", err)
return
}
// Step 3: Generate HMAC signature using SHA-256
h := hmac.New(sha256.New, []byte(apiSecret))
h.Write(payloadJSON)
generatedSignature := hex.EncodeToString(h.Sum(nil))
// Step 4: Verify the signature
if hmac.Equal([]byte(generatedSignature), []byte(receivedSignature)) {
fmt.Println("Signature is valid!")
} else {
fmt.Println("Signature is invalid!")
}
}
import java.security.MessageDigest
import javax.crypto.Mac
import javax.crypto.spec.SecretKeySpec
import java.util.*
import com.fasterxml.jackson.databind.ObjectMapper
fun main() {
// Payload received from webhook
val payload = mapOf(
"status" to "failed",
"type" to "sale",
"amount" to "55.00",
"reference" to "DazuknyVyG",
"transaction_id" to "A49dfkqvw",
"payment_method" to "Card (VISA)",
"created_at" to "2025-02-25 05:18:56",
"processor_name" to "Trust Payments",
"currency" to "USD"
)
// Fetch API Secret Text from settings/config
val apiSecret = System.getenv("API_SECRET") ?: "default_secret" // Replace with your actual config fetching logic
// Received signature (from the request headers or body)
val receivedSignature = "your_received_signature_here" // Replace with the actual signature
// Step 1: Sort the payload by keys alphabetically
val sortedPayload = payload.toSortedMap()
// Step 2: Convert payload to JSON string
val objectMapper = ObjectMapper()
val payloadJson = objectMapper.writeValueAsString(sortedPayload)
// Step 3: Generate HMAC signature using SHA-256
val generatedSignature = generateHmacSha256(payloadJson, apiSecret)
// Step 4: Verify the signature
if (generatedSignature == receivedSignature) {
println("Signature is valid!")
} else {
println("Signature is invalid!")
}
}
// Function to generate HMAC-SHA256 signature
fun generateHmacSha256(data: String, secret: String): String {
val mac = Mac.getInstance("HmacSHA256")
val secretKeySpec = SecretKeySpec(secret.toByteArray(Charsets.UTF_8), "HmacSHA256")
mac.init(secretKeySpec)
val hmacBytes = mac.doFinal(data.toByteArray(Charsets.UTF_8))
return bytesToHex(hmacBytes)
}
// Helper function to convert byte array to hex string
fun bytesToHex(bytes: ByteArray): String {
val hexString = StringBuilder()
for (b in bytes) {
hexString.append(String.format("%02x", b))
}
return hexString.toString()
}
Merchant Account Id
merchantAccountId :
And use the "Account No" as "merchantAccountId" from the "Api Config" menu.
See the attached image
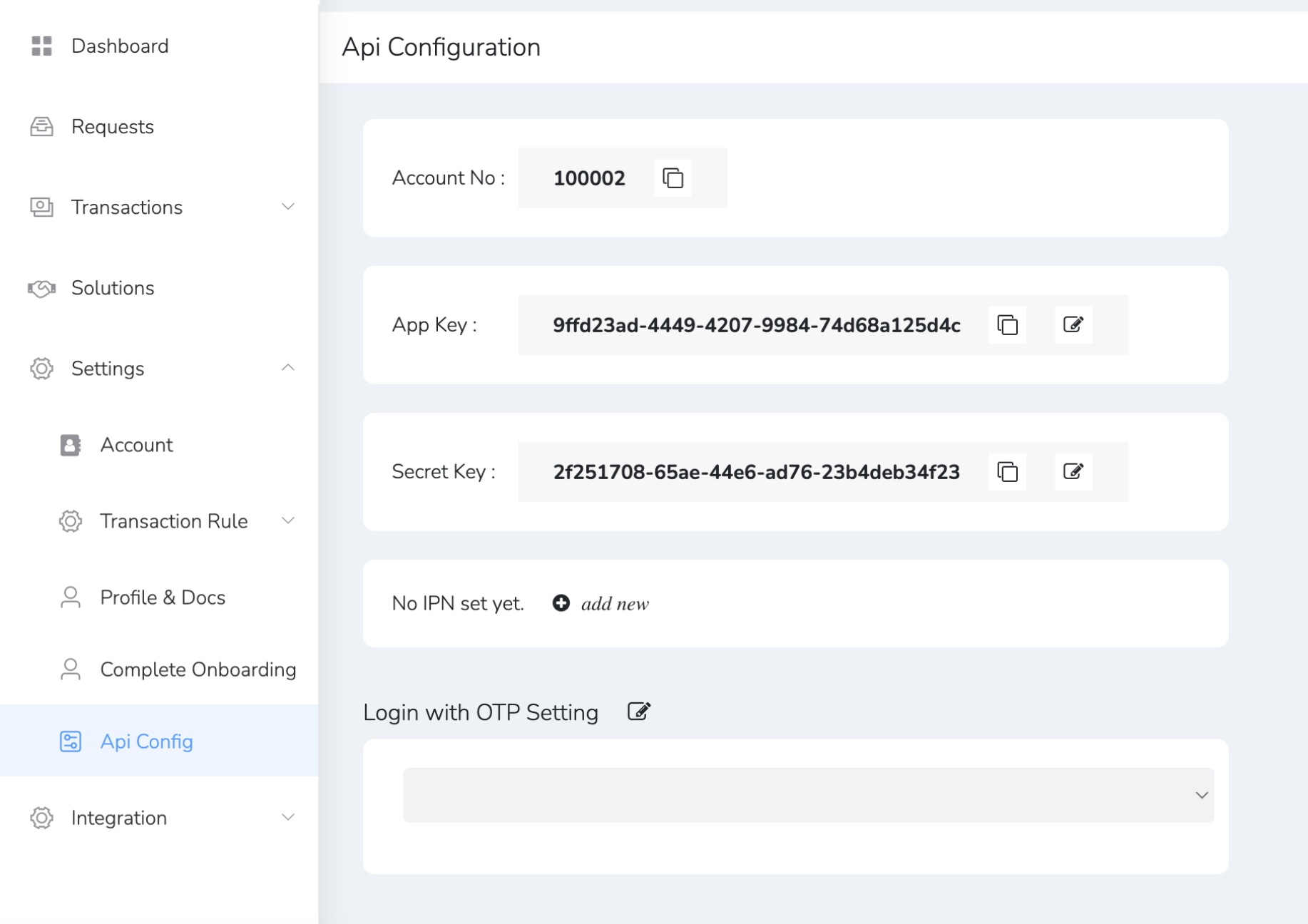
Set your "IPN (Instant Payment Notification)" url for get transactions callback response.
Redirect URL's
Url's :
Here you can find "success url", "failed url" for redirect to actual urls after transaction.
See the attached image
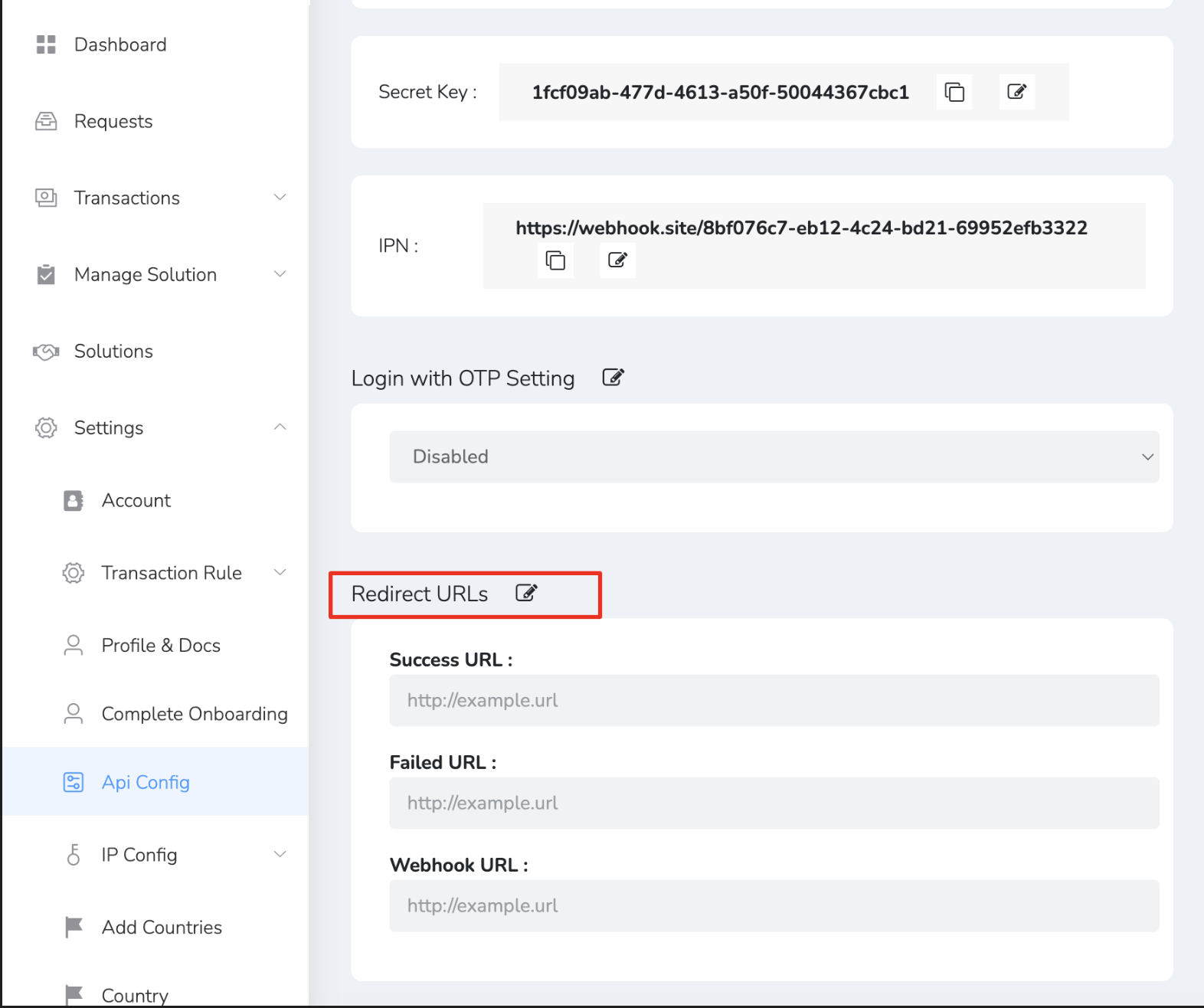
Enable Solution
In order to enable various solutions you have to navigate over to "Select Solutions ->", once you try to enable them you will be prompted to input the required credentials that each solution provider requests.
Once you have entered the credentials you need to change "Status -> Active" and then click the "Save" button.
See the attached image
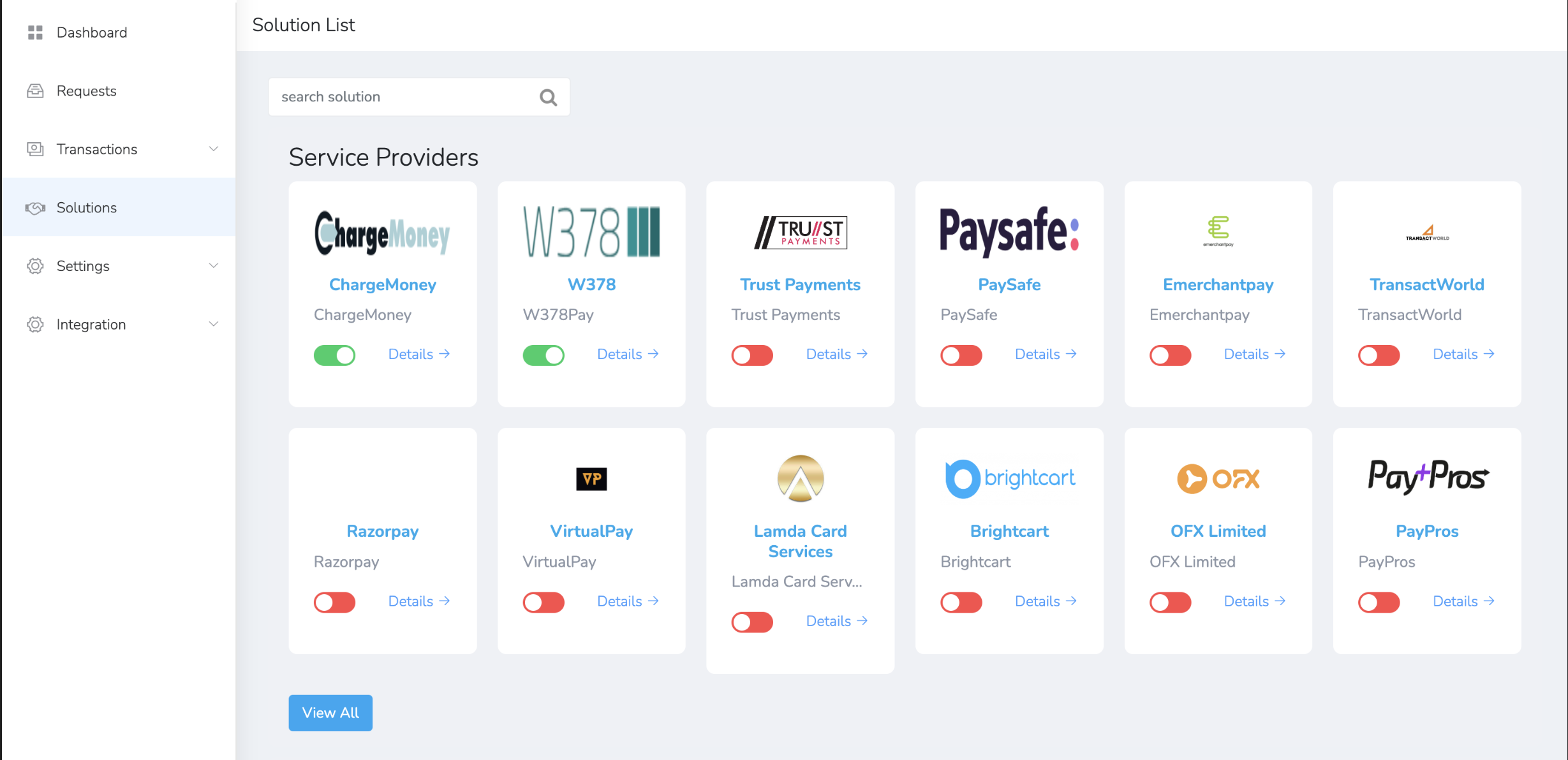
CLick to Enable button then filled all required field and Save.
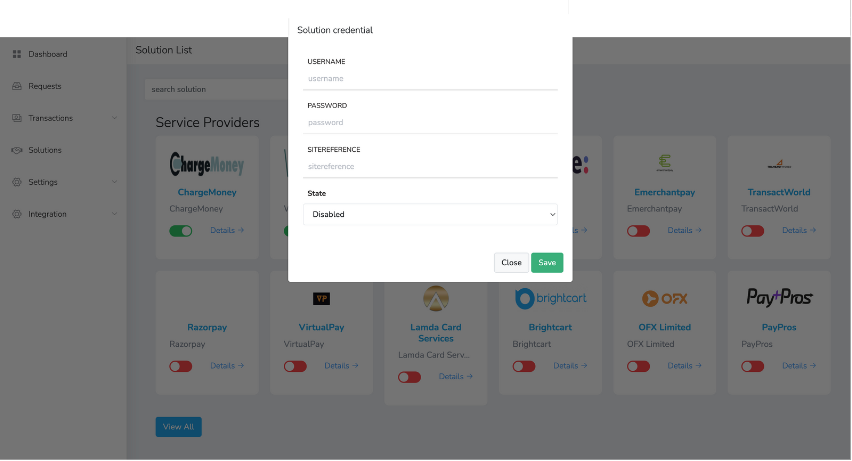
Test Cards
Here is some dummy card details.
Success Cards :
4000000000000077
4111111111111111
4242424242424242
Decline Cards :
4917484589897107
CVV : 123
Date : 02/2029
Payout
This section explains about Payout.
Bank Payout
Name: Make Bank Payouts
Method : POST
Url : {{base_url}}/api/v1/payout/process
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Body
Raw JSON
{
"basicProfile": {
"country": "Sweden",
"dob": "2024-04-01", // format must be yyyy-mm-dd
"firstName": "John",
"lastName": "Doe",
"email": "[email protected]",
"contactNumber": "9034893274",
"postcode": "11115",
"premise": "Stockholmes",
"street": "Stockholmes",
"state": "Dalarna County",
"town": "Borlänge"
},
"processorRequiredFields": {
"accountName": "John Doe",
"accountNumber": "5648915103",
"bankName": "SIAM COMMERCIAL BANK PUBLIC COMPANY LTD.",
"bankCode": "014"
},
"reference": "papaya-123q", // 15 characters or less
"merchantAccountId": "{{merchantAccountId}}",
"amount": "10",
"currency": "THB", // ISO 4217 format
"solutionId": "{{solutionId}}", // Id from Solution list
"payoutType": "bankPayout"
}
Response Example
JSON
{
"success": true,
"message": "Bank Payout Processed",
"data": {
"success": true,
"reference_id": "55-DuqXNBv0Q7",
"redirect_to": null
}
}
Card Payout
Name: Make Card Payouts
Method : POST
Url : {{base_url}}/api/v1/payout/process
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Body
Raw JSON
{
"basicProfile": {
"country": "Sweden",
"dob": "2006-07-09", // format must be yyyy-mm-dd
"firstName": "John",
"lastName": "Doe",
"email": "[email protected]",
"contactNumber": "9034893274",
"postcode": "11115",
"premise": "Stockholmes",
"street": "Stockholmes",
"state": "Gävleborg County",
"town": "Alfta"
},
"processorRequiredFields": {
"expiryDate": "02/2025",
"card": "4111111111111111",
"cardName": "John Doe",
"secret": "123"
},
"reference": "IC-Payout-0011",
"merchantAccountId": "{{merchantAccountId}}",
"amount": "50.00",
"currency": "USD", // ISO 4217 format
"solutionId": "{{solutionId}}", // Id from Solution list
"payoutType": "cardPayout"
}
Response Example
JSON
{
"success": true,
"message": "Card Payout Processed",
"data": {
"success": true,
"reference_id": "11-DuqXNBv0Q2",
"redirect_to": null
}
}
Get Solution List
Name: Get Solution List by Merchant Account ID
Method : GET
Url : {{base_url}}/api/v1/merchant/active/solutions?payout_type=bankPayout&merchantAccountId={{merchantAccountId}}
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Param
merchantAccountId : your_account_no
payout_type : bankPayout // or cardPayout
Response Example
JSON
{
"success": true,
"message": "Success",
"data": [
{
"id": "269b8d4a-151e-4f17-b3f0-050277618ac2", // this Solution ID is required in other requests
"name": "Trust Payments",
"logo": "https://centralisera-staging.s3.amazonaws.com/solution/logo/63d134c84edb5490.jpg",
"supported_currencies": [
"USD",
"EURO"
],
"regions": [
"Africa"
],
"dynamic_currency_supported": 1
}
]
}
Server to Server
This section explains about Server to Server Payments.
Direct Server to Server Payment
Name: Make Direct Server to Server Payments
Method : POST
Url : {{base_url}}/api/v1/payment/process-transaction
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Body
Raw JSON
{
"firstName": "John",
"middleName": "", // optional
"lastName": "Doe",
"reference": "DuqXNBv0Q", // must be more than 10 characters and special characters are not allowed
"email": "[email protected]",
"dob": "1990-01-01", // format must be yyyy-mm-dd
"contactNumber": "+12345678",
"address": "SE",
"country": "Sweden",
"state": "N/A",
"city": "N/A",
"zipCode": "1212",
"currency": "USD", // ISO 4217 format
"amount": "55.00",
"ttl": 5,
"tagName": "VIP Tag",
"webhookUrl": "", // optional
"productUrl": "", // optional
"redirectUrl": "", // optional
"merchantAccountId": "{{merchantAccountId}}",
"solutionUniqueId": [],
"card": "{{test_card}}",
"secret": "123",
"expiry_date": "06/24",
"card_name": "MD X",
"customerBrowserUserAgent": "Mozilla 52.0",
"customerIP": "1.1.1.1"
}
2D Success Response Example
JSON
{
"success": true,
"message": "Payment Request Processed",
"data": {
"success": true,
"reference_id": "11-DuqXNBv0Q2",
"redirect_to": null
}
}
3D Redirect URL Response Example
JSON
{
"success": true,
"message": "Payment Request Failed",
"data": {
"success": true,
"reference_id": "11-DuqXNBv0Q",
"redirect_to": "https://staging.centralisera.com/three_d/acs/redirect?payment_id=11-DuqXNBv0Q"
}
}
Auth Error Response Example
JSON
{
"success": false,
"message": "Merchant Authentication failed",
"errors": {
"Auth": [
"Merchant Authentication failed"
]
}
}
Paymid Recurring
This section explains about Recurring Payment Requests.
Recurring Payment
Name: Payment Request with Recurring Enable
Method : POST
Url : {{base_url}}/api/v1/payment/request
Description : If there is a trial/initial period before the recurring payment starts, then trialAmount and trialInterval should be added. For example: if trialAmount is 10 and trialInterval is 3 then first payment will be 10 and after 3 days, the actual recurring payment of 50 will occur each 30 days.
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Body
Raw JSON
{
"firstName": "John",
"middleName": "", // optional
"lastName": "Doe",
"reference": "DuqXNBv0QQ-13",
"email": "[email protected]",
"dob": "1990-01-01", // format must be yyyy-mm-dd
"contactNumber": "+12345678",
"address": "SE",
"country": "Sweden",
"state": "N/A",
"city": "N/A",
"zipCode": "1212",
"currency": "USD", // ISO 4217 format
"amount": "55.00",
"ttl": 5,
"tagName": "VIP Tag",
"webhookUrl": "", // optional
"productUrl": "", // optional
"redirectUrl": "", // optional
"merchantAccountId": "{{merchantAccountId}}",
"solutionUniqueId": [],
"isRecurring": 1,
"recurringInterval": 30,
"recurringAmount": 50,
"trialAmount": 10, // optional
"trialInterval": 3, // optional
}
Response Example
JSON
{
"success": true,
"message": "Payment Request Accepted",
"data": {
"payment_url": "https://sgw.paymid.com/checkout-page/38e65db8-5934-48a8-a569-4038c50d1b23"
}
}
Subscription List
Name: Get Subscription List by Merchant Account ID
Method : GET
Url : {{base_url}}/api/v1/subscriptions/get-list?merchantAccountId={{merchantAccountId}}&page=1&status=active
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Param
merchantAccountId : your_account_no
page : 1 // optional
status : active // active or inactive or cancelled
Response Example
JSON
{
"success": true,
"message": "success.",
"data": [
{
"subscription_id": "209de9e7-c4d0-400e-b463-928ca1d6343a",
"amount": "55",
"status": "active",
"last_payment_status": "success",
"start_date": "2024-04-25 10:23:37"
},
{
"subscription_id": "491e66ee-a8bb-4b2b-bcb3-eb95fe787924",
"amount": "55",
"status": "active",
"last_payment_status": "success",
"start_date": "2024-05-02 15:18:34"
},
{
"subscription_id": "8af65bbc-b160-4e32-8909-f5b21d0d8852",
"amount": "55",
"status": "active",
"last_payment_status": "success",
"start_date": "2024-05-13 19:06:14"
},
{
"subscription_id": "03cdef06-1ae1-44c0-b674-eabdf30f6c20",
"amount": "55",
"status": "active",
"last_payment_status": "success",
"start_date": "2024-06-21 19:42:28"
}
],
"pagination": {
"total": 4,
"current_items_count": 4,
"items_per_page": 10,
"current_page_no": 1,
"last_page_no": 1,
"has_more_pages": false
}
}
Transaction List
Name: Get Transaction List by Merchant Account ID
Method : GET
Url : {{base_url}}/api/v1/subscriptions/get-list?merchantAccountId={{merchantAccountId}}&page=1&status=active
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Param
merchantAccountId : your_account_no
page : 1 // optional
status : active // active or inactive or cancelled
Response Example
JSON
{
"success": true,
"message": "success.",
"data": [
{
"subscription_id": "209de9e7-c4d0-400e-b463-928ca1d6343a",
"amount": "55",
"status": "active",
"last_payment_status": "success",
"start_date": "2024-04-25 10:23:37"
},
{
"subscription_id": "491e66ee-a8bb-4b2b-bcb3-eb95fe787924",
"amount": "55",
"status": "active",
"last_payment_status": "success",
"start_date": "2024-05-02 15:18:34"
},
{
"subscription_id": "8af65bbc-b160-4e32-8909-f5b21d0d8852",
"amount": "55",
"status": "active",
"last_payment_status": "success",
"start_date": "2024-05-13 19:06:14"
},
{
"subscription_id": "03cdef06-1ae1-44c0-b674-eabdf30f6c20",
"amount": "55",
"status": "active",
"last_payment_status": "success",
"start_date": "2024-06-21 19:42:28"
}
],
"pagination": {
"total": 4,
"current_items_count": 4,
"items_per_page": 10,
"current_page_no": 1,
"last_page_no": 1,
"has_more_pages": false
}
}
Cancel Subscription
Name: Cancel Subscription by Merchant Account ID
Method : POST
Url : {{base_url}}/api/v1/subscriptions/cancel
Authorization
Basic Auth
Username : App Key
Password : Secrete Key
Body
Raw JSON
{
"merchantAccountId": "{{merchantAccountId}}",
"subscription_id": "03cdef06-1ae1-44c0-b674-eabdf30f6c20" // Actual subscription id from your list
}
Response Example
JSON
{
"success": true,
"message": "success.",
"data": {
"subscription_id": "03cdef06-1ae1-44c0-b674-eabdf30f6c20",
"status": "cancelled"
}
}